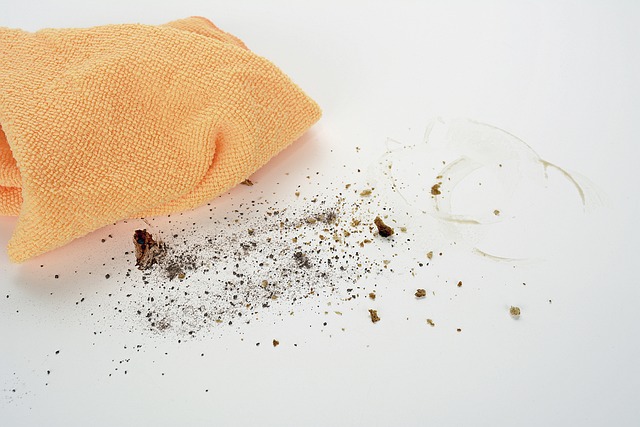
เรื่องต้องรู้ของผ้าไมโครไฟเบอร์
หากว่าเอ่ยถึงผ้าไมโครไฟเบอร์ หลายๆ คนคงคุ้นหูกันเป็นอย่างดี เนื่องจากว่าผ้าชนิดนี้เป็นผ้าที่ได้รับความนิยมมาเป็นเวลานาน อีกทั้งยังตอบโจทย์การทำความสะอาดในทุกๆ ด้าน อย่างไรก็ดีใครที่ชื่นชอบการล้างหรือการเช็ดทำความสะอาดของตนเอง มาดูพร้อมๆ กันดีกว่าหรือไม่ว่าผ้าไมโครไฟเบอร์นั้นมีเรื่องอะไรที่ต้องรู้บ้าง
คุณสมบัติของผ้าชนิดไมโครไฟเบอร์
ผ้าชนิดนี้มีคุณสมบัติในการเช็ดคราบน้ำต่างๆ ได้อย่างแห้งสนิท และไม่เหลือคราบใดๆ ซึ่งผ้าชนิดนี้เป็นผ้าเส้นใยสังเคราะห์ที่ผลิตจากเส้นใยสองประเภท ได้แก่ เส้นใยโพลีเอสเตอร์ ซึ่งมีคุณสมบัติของไฟฟ้าสถิต ซึ่งจุดเด่นของการกักเก็บฝุ่นได้เป็นอย่างดีที่สุด ในขณะที่มีโพลิเอไมด์อันเป็นคุณสมบัติในการดูดซับสิ่งสกปรก โดยสามารถเก็บได้ทั้งคราบสกปรก และคราบมันบนพื้นผิวโดยที่ไม่ต้องสร้างรอยใดๆ เลย อย่างไรก็ดีคุณสมบัติโดยรวมของไมโครไฟเบอร์จะเน้นการทำความสะอาดที่มีประสิทธิภาพ
- เส้นใย Polyester สำหรับผ้าชนิดนี้จะใช้สัดส่วนของโพลิเอสเตอร์ต่อโพลิเอไมด์ในคุณสมบัติ 80 : 20 ซึ่งมีค่าไฟฟ้าสถิตย์ เมื่อใช้ผ้าชนิดไมโครไฟเบอร์เช็ดแล้วจะทำให้พื้นผิวแห้งจนกระทั่งเกิดไฟฟ้าสถิตขึ้น เนื่องจากว่าเส้นใยมีขนาดที่เล็กและละเอียด ทำให้คราบสกปรกรวมไปถึงฝุ่นผงต่างๆ มีขนาดเล็ก โดยเกาะติดไปกับพื้นผิวของผ้าไมโครไฟเบอร์ โดยฝุ่นเองก็จะไม่ฟุ้งและไม่ทำให้เกิดคราบใดๆ
- เส้นใย Polyamide สำหรับผู้ที่ต้องการให้ผ้านุ่มกว่าแบบแรกก็ให้เลือกเป็นผ้าโพลิเอไมด์ ซึ่งสัดส่วนจะอยู่ที่ 70:30 โดยจะมีความสามารถในการดูดซับน้ำ ทำความสะอาดผิวที่เปียกและชื้นได้ดีกว่าเส้นใยธรรมชาติทั่วไป ซึ่งจะทำให้การลดคราบสกปรก คราบไขมันต่างๆ และคราบแบคทีเรีย โดยพื้นผิวของวัสดุมีประสิทธิภาพไม่ทำให้เกิดคราบน้ำและรอยด่าง รวมไปถึงรอยขีดข่วน และสามารถกำจัดคราบสกปรกได้เกือบ 100 เปอร์เซ็นต์ และลดแบคทีเรียได้เกือบ 100 เปอร์เซ็นต์ และจะใช้ได้หลากหลายพื้นผิวไม่ว่าจะเป็นเมลามีน เครื่องหนัง ผิวโลหะ เป็นต้น
ประโยชน์
สำหรับผ้าชนิดนี้มักนำมาทำความสะอาดพื้นผิวช่วยลดการเกิดริ้วรอย ทั้งยังเบาแรงของคนที่ทำความสะอาด ไม่เป็นขน อีกทั้งยังเช็ดได้ลื่น และซับน้ำได้อย่างดีเยี่ยม สามารถขจัดคราบสกปรกให้สะอาดในการเช็ดแค่เพียงครั้งเดียว
จะเห็นได้อย่างชัดเจนเลยว่าการเลือกสิ่งที่ดีที่สุดในการทำความสะอาดบ้านคือการใช้ผ้าไมโครไฟเบอร์ และควรเลือกซื้อเผื่อเอาไว้หลายๆ ผืนเพื่อการใช้ประโยชน์ได้อย่างแท้จริง